The API Call node allows you to interact with external APIs directly from your workflow. Whether you need to fetch data, submit information, or integrate with third-party services, this node provides a powerful and flexible way to make HTTP requests.
Key Features #
- Supports all standard HTTP methods (GET, POST, PUT, DELETE, etc.)
- Customizable headers and query parameters
- Multiple authentication options
- Response caching capabilities
- JSONPath support for data extraction
- Dynamic input support from previous nodes
- Automatic retry on failure
- Secure credential handling
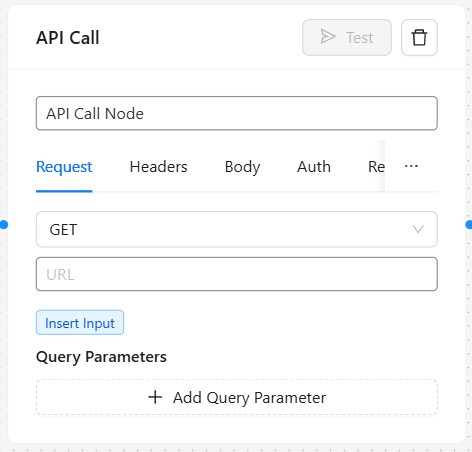
Basic Configuration #
URL and Method #
- URL: Enter the API endpoint URL
- Method: Select from GET, POST, PUT, DELETE, PATCH, HEAD, or OPTIONS
- Query Parameters: Add key-value pairs that will be appended to the URL
- You can use the “Insert Input” feature to dynamically set parameter values from previous nodes
Headers #
Configure request headers by adding name-value pairs. Common headers include:
Content-Type: application/json
Accept: application/json
Request Body #
For POST, PUT, and PATCH requests, you can include a request body:
- Plain text
- JSON data
- Dynamic content from previous nodes
Authentication #
The node supports multiple authentication methods:
No Auth #
Use for public APIs or when authentication is not required.
Basic Auth #
- Username and password authentication
- Credentials are automatically encoded and sent in the Authorization header
Bearer Token #
- Common for OAuth 2.0 implementations
- Simply provide your token, and it will be sent as “Bearer {token}”
API Key #
- Specify both the API key name and value
- The key will be sent as a header (e.g., “X-API-Key: your-key-here”)
Advanced Features #
Response Configuration #
Timeout Settings #
- Set custom timeout duration (1-300 seconds)
- Default: 30 seconds
Retry Logic #
- Configure retry attempts (0-5)
- Automatic exponential backoff
- Only retries on server errors (5xx) or network issues
JSONPath Extraction #
Extract specific data from JSON responses using JSONPath syntax:
$.data.items[*].name
– Extract all item names from an array$.user.email
– Extract user email$..price
– Extract all prices from any level
Response Caching #
Optimize performance by caching responses:
- Enable/disable caching
- Set cache duration (60-86400 seconds)
- Automatic cache invalidation
Using Dynamic Inputs #
The API Call node can use outputs from previous nodes in various ways:
- Click the “Insert Input” button next to any field
- Select the source node and specific output
- The input will be inserted as a tag like
[Input from Node-1234]
Common use cases for dynamic inputs:
- Using authentication tokens from previous API calls
- Building URLs dynamically
- Including data from previous nodes in the request body
Example Use Cases #
1. Weather API Integration #
Method: GET
URL: https://api.weatherapi.com/v1/current.json
Query Parameters:
- key: your-api-key
- q: [Input from Location-Node]
2. CRM Data Update #
Method: POST
URL: https://api.crm.com/v1/contacts
Headers:
- Content-Type: application/json
- Authorization: Bearer [Input from Auth-Node]
Body: {
"name": [Input from Form-Node],
"email": [Input from Form-Node],
"status": "active"
}
Best Practices #
- Error Handling
- Use retry settings for unreliable APIs
- Extract specific error messages using JSONPath
- Consider adding condition nodes after API calls
- Security
- Never hardcode sensitive credentials
- Use environment variables when possible
- Enable SSL verification
- Performance
- Enable caching for frequent, unchanged requests
- Set appropriate timeouts
- Use JSONPath to extract only needed data
- Maintenance
- Document API endpoints and requirements
- Use meaningful node names
- Test API calls before running workflows
Troubleshooting #
Common issues and solutions:
- Authentication Failures
- Verify credentials are correct
- Check if token has expired
- Ensure correct auth type is selected
- Timeout Errors
- Increase timeout duration
- Enable retry attempts
- Check API service status
- Data Extraction Issues
- Verify JSONPath syntax
- Check response format
- Use test button to validate extraction
- Invalid Responses
- Validate request format
- Check content-type headers
- Verify API documentation requirements